The Trigger Action tests an incoming Event against a set of predefined rules. These rules determine the flow of the story by comparing an incoming value against a test value.
Some common use-cases for the Trigger Action include:
Ignoring Events that don't require processing
Send Events downstream for additional analysis
Further process events that are sent from a particular application
Rule types
matches regex
: The incoming value matches a defined regular expression.does not match regex
: The incoming value doesn't match a defined regular expression.is equal to
: The incoming value is equal to the test value.is not equal to
: The incoming value is not equal to the test value.is less than
: The incoming (numeric) value is less than the test value.is less than or equal to
: The incoming (numeric) value is less than or equal to the test value.is greater than
: The incoming (numeric) value is greater than the test value.is greater than or equal to
: The incoming (numeric) value is greater than or equal to the test value.contains
: The incoming value contains the test value. If the incoming value is an array, the test value must match one of the items in that array. If the incoming value is text, the test value must be found within the incoming text.does not contain
: The incoming value does not contain the test value. If the incoming value is an array, the test value must not match any of the items in that array. If the incoming value is text, the test value must not be found within the incoming text.formula is true
: The result of the formula expression is "truthy".formula is false
: The result of the formula expression is "falsy".
"Truthy" and "falsy" values
Every value in Tines can be categorized as either "truthy" or "falsy". "Falsy" values include FALSE
, NULL
, and an empty array, object or text string ([]
, {}
or ""
). "Truthy" values are everything else. formula is true
matches "truthy" values and formula is false
matches "falsy" values. If you want the rule to only match TRUE
or FALSE
, use is equal to
.
Configuration Options
rules
: The rules array contains sets oftype
,path
andvalue
fields.type
:regex
,!regex
,field==value
,field!=value
,field<value
,field<=value
,field>value
,field>=value
,in
,not in
,formula
ornot formula
path
: Specify the incoming value, used as the left side of the comparison. Can be a path to a value or a more complex formula.value
: Specify the test value, used as the right side of the comparison.
must_match
: (Optional) By default, all rules must match for the Action to trigger an Event emit. You can switch this so that only one rule must match by settingmust_match
to '1'.emit_no_match
: (Optional) By settingemit_no_match
totrue
an event will also be emitted if the rules do not match.
No match branch
If instead of halting the story run you'd like to redirect it depending on a rule, you can use the no match branch to send the story in a different direction depending on the result. This is a useful pattern, equivalent to an "if/else" flow in a software language.
Emitted Events
When a rule is matched, Events emitted by the Trigger Action will contain a field named rule_matched
set to "true".
{
"rule_matched": true
}
When a rule is not matched and emit_no_match
is configured, Events will be emitted by the Trigger Action and will contain a field named rule_matched
set to "false".
If emit_no_match
is not configured and a rule is not matched, these Events will still be emitted, but they will be labeled No match
.
{
"rule_matched": false
}
Example Configuration Options
Emit an Event when the contents of the 'size' field in an incoming Events are greater than 5.
{
"rules": [
{
"type": "field>value",
"path": "<<size>>",
"value": "5"
}
]
}
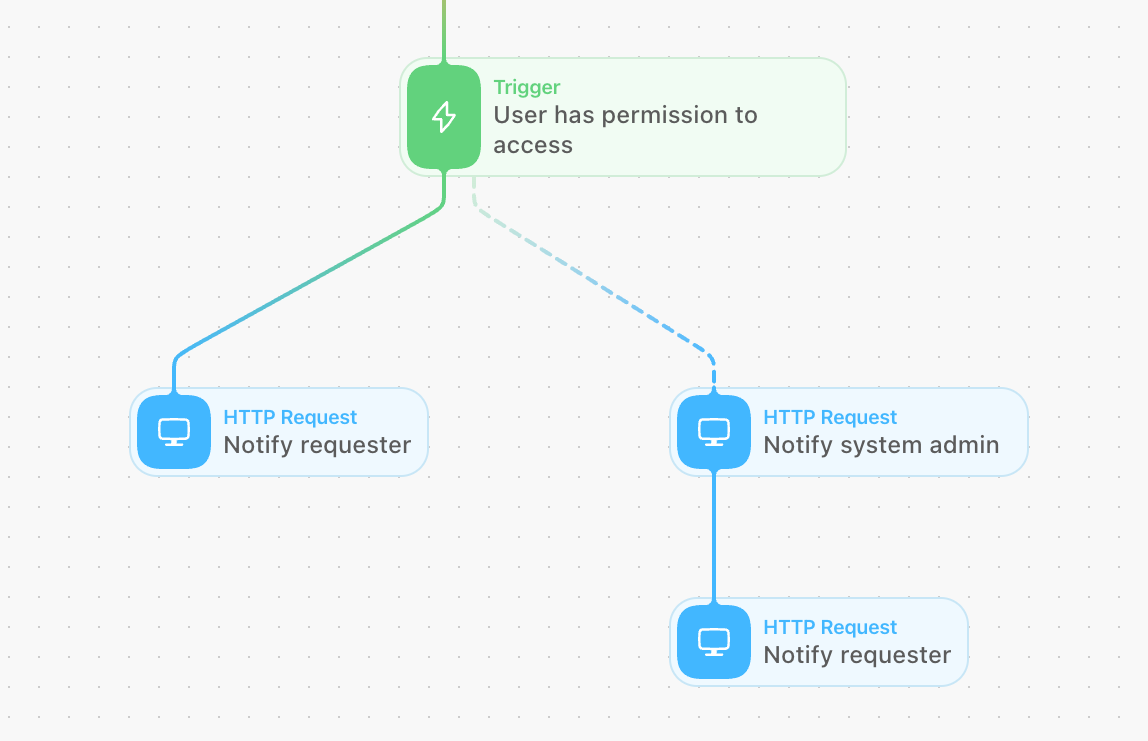
Emit an Event when the content of the 'body' field contain an email address.
{
"rules": [
{
"type": "regex",
"path": "<<body>>",
"value": "\\b[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\\.[a-zA-Z]{2,4}\\b"
}
]
}
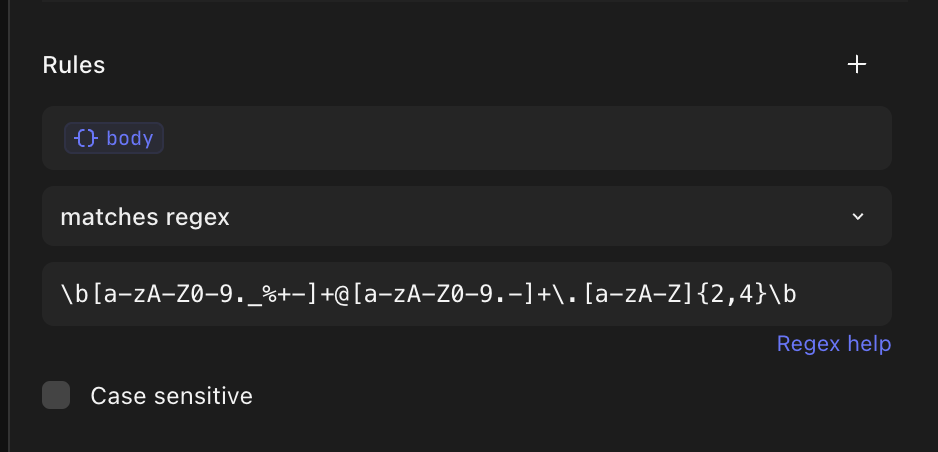
Emit an event when 'dog' is found in the array of animal types listed in path
.
{
"rules": [
{
"type": "in",
"path": ["dog", "bird", "turtle"],
"value": "dog"
}
]
}
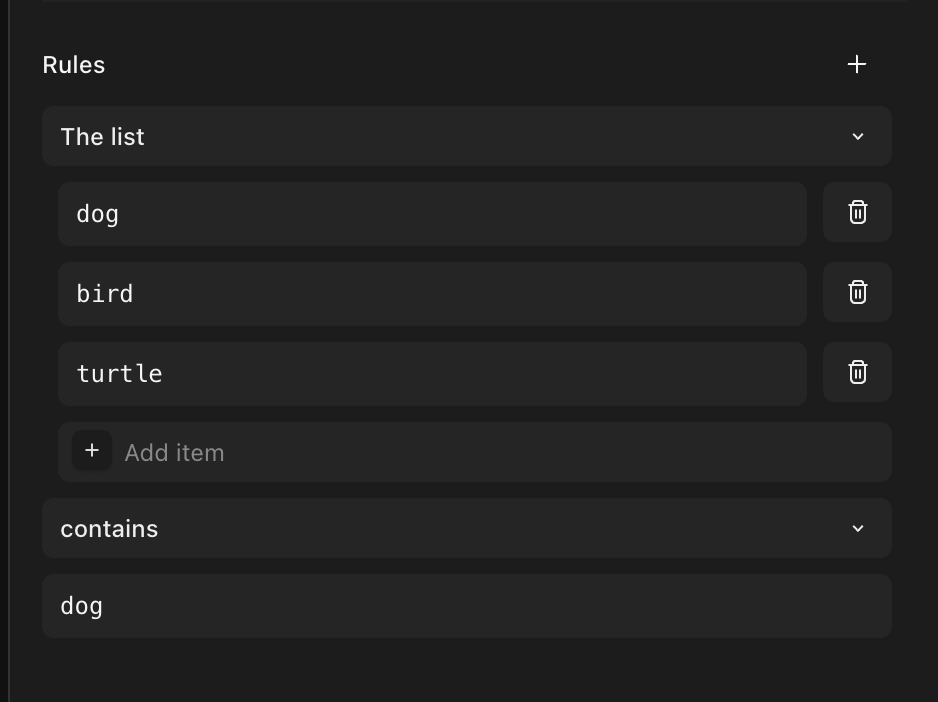
Value can also be an array. In this case the action will emit an event when either 'dog' or 'cat' is found in the array of animal types listed in path
.
{
"rules": [
{
"type": "in",
"path": ["dog", "bird", "turtle"],
"value": ["dog", "cat"]
}
]
}
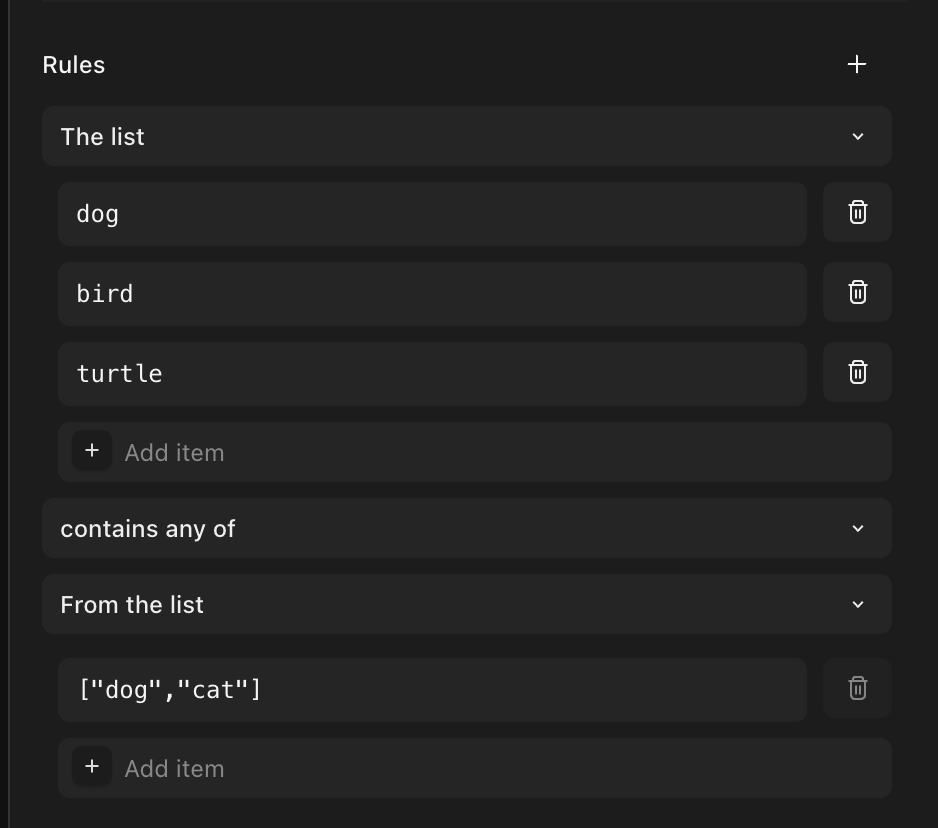
Emit an Event when 'dog' is not contained in the array of animal types listed in path
.
{
"rules": [
{
"type": "not in",
"path": ["bird", "turtle"],
"value": "dog"
}
]
}
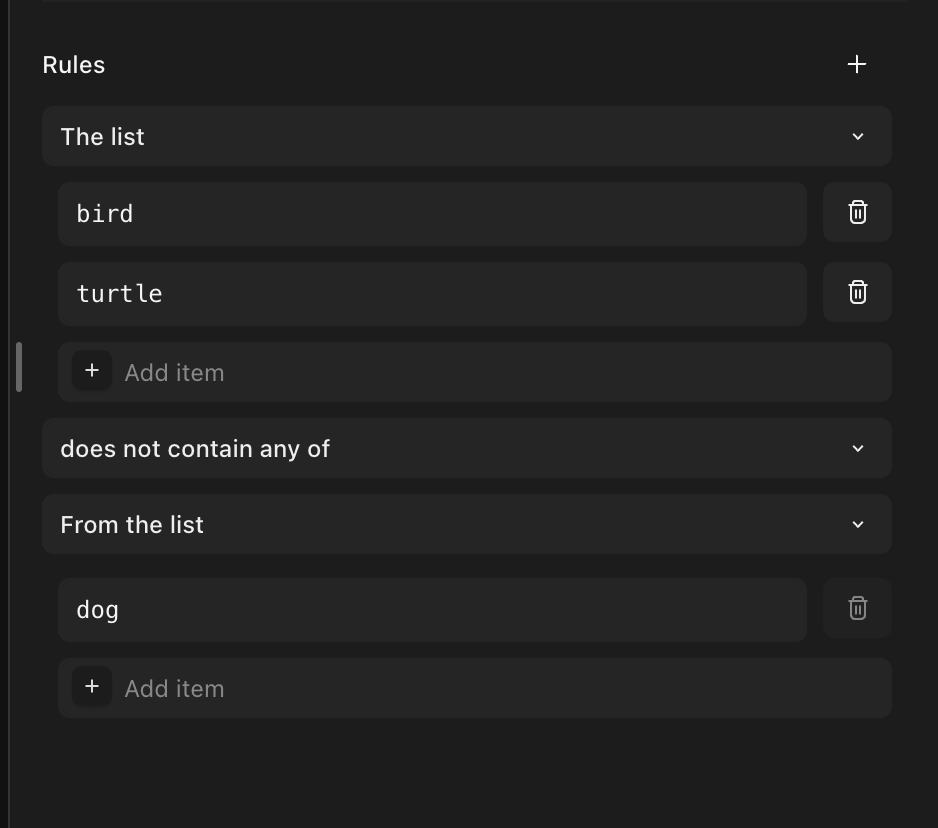
Value can also be an array. In this case it means that neither 'dog' nor 'cat' are contained in the array of animal types listed in path
.
{
"rules": [
{
"type": "not in",
"path": ["bird", "turtle"],
"value": ["dog", "cat"]
}
]
}
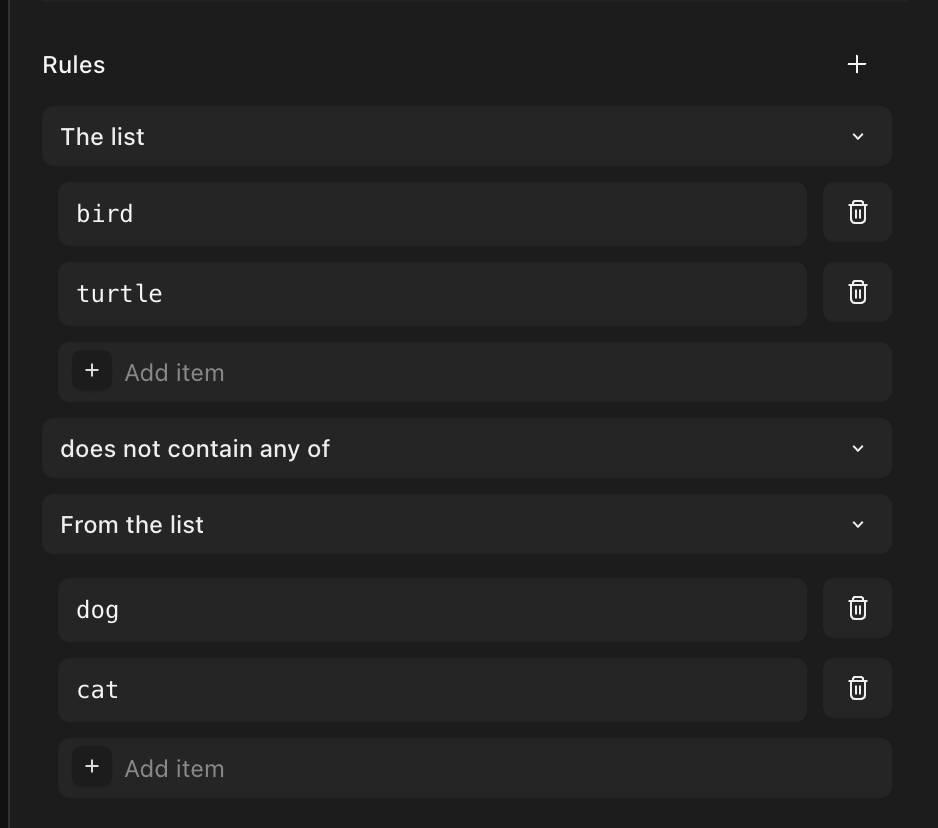
Emit an Event when the contents of the 'size' field of an incoming Event is greater than 0 AND the contents of the 'username' field is "alice".
{
"rules": [
{
"type": "field>value",
"path": "<<size>>",
"value": 0
},
{
"type": "field==value",
"path": "<<username>>",
"value": "alice"
}
]
}
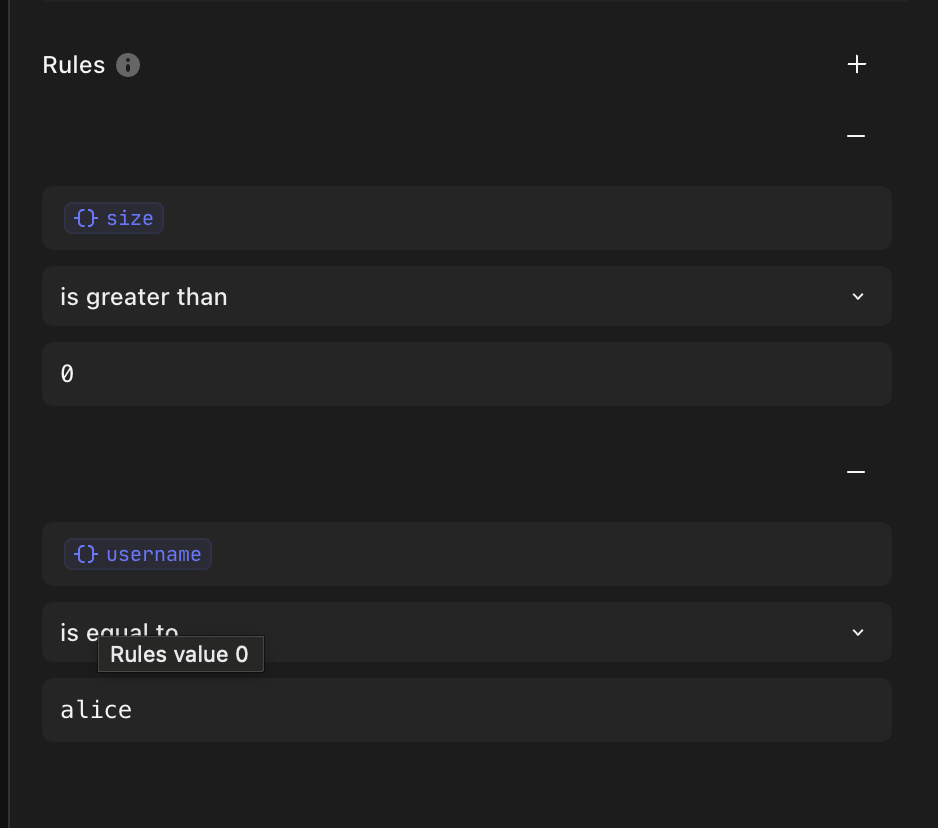
Emit an Event when the contents of the 'size' field of an incoming Event is greater than 0 OR the contents of the 'username' field is "alice".
{
"rules": [
{
"type": "field>value",
"path": "<<size>>",
"value": 0
},
{
"type": "field==value",
"path": "<<username>>",
"value": "alice"
}
],
"must_match": 1
}
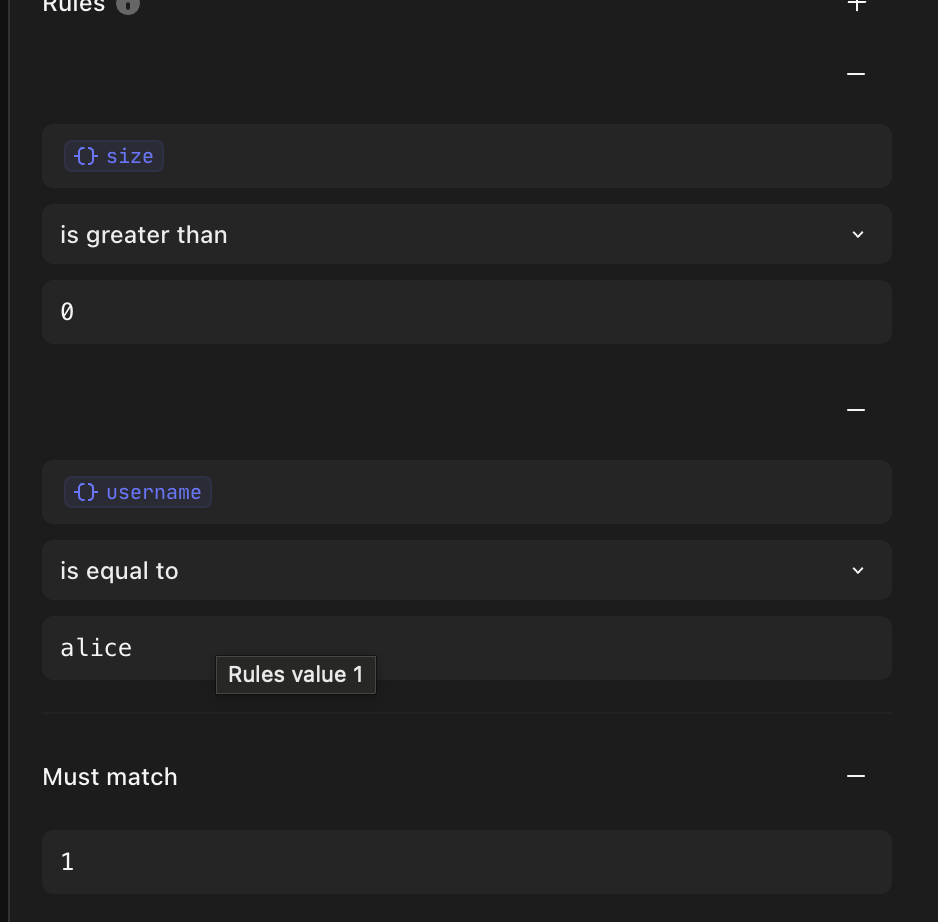
When receiving the following event:
{
"student": {
"name": "Alice",
"age": "20"
}
}
Emit an event if the student is over 25 years of age:
{
"rules": [
{
"type": "field>value",
"value": "25",
"path": "<<student.age>>"
}
]
}
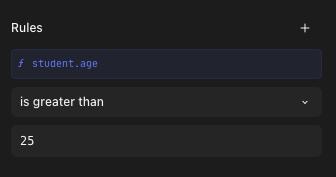